반응형
형식 변환은 크게 묵시적(암시적) 형변환과 명시적 형변환으로 구분할 수 있습니다. 묵시적 형변환은 변환 형식이 안전하게 유지됩니다. 데이터가 손실되지 않는 특성으로 인해 특수한 구문이 필요치 않습니다. 예를 들어 숫자 형식 중 int는 그보다 큰 long 형식에 그대로 담을 수 있습니다.
Data TypeSize (bytes)Range
byte | 1 | 0..255 |
sbyte | 1 | -128..127 |
short | 2 | -32,768 .. 32,767 |
ushort | 2 | 0 .. 65,535 |
int | 4 | -2,147,483,648 .. 2,147,483,647 |
uint | 4 | 0 .. 4,294,967,295 |
long | 8 | -9,223,372,036,854,775,808 .. 9,223,372,036,854,775,807 |
ulong | 8 | 0 .. 18,446,744,073,709,551,615 |
float | 4 | -3.402823e38 .. 3.402823e38 |
double | 8 | -1.79769313486232e308 .. 1.79769313486232e308 |
decimal | 16 | -79,228,162,514,264,337,593,543,950,335 .. 79,228,162,514,264,337,593,543,950,335 |
bool | 1 | True or False |
char | 2 | A unicode character (0 .. 65,535) |
묵시적 형변환
short shortNumber = 23;
// Implicit conversions
int intNumber = shortNumber;
float someFloat = shortNumber;
double firstDouble = intNumber;
double secondDouble = shortNumber;
묵시적 형변환 테이블
From To
sbyte short int long float double decimal
byte short ushort int uint long ulong float double decimal
short int long float double decimal
ushort int uint long ulong float double decimal
int long float double decimal
uint long ulong float double decimal
long ulong float double decimal
float double
char ushort int uint long ulong float double decimal
묵시적 형변환 그래프
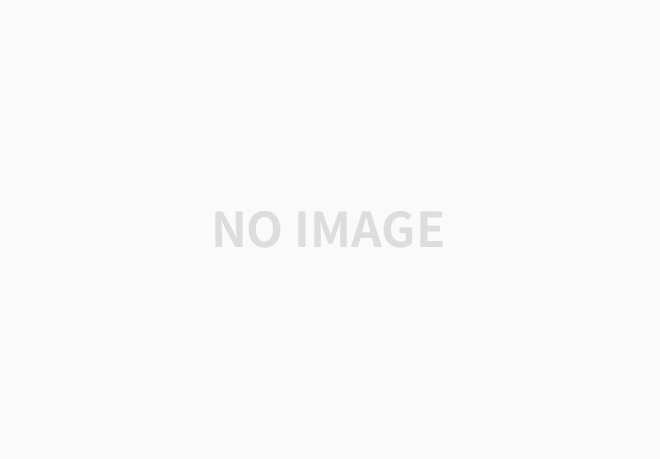
명시적 형변환
long을 int에 저장하려면 다음처럼 명시적으로 (int) 구문을 붙여서 형변환해야합니다.
명시적 형변환은 Casting이라고도 합니다.
이 경우에는 데이터가 손실될 수 있습니다. 에러가 발생하지 않지만 잘못된 값이 저장되는 경우를 봅시다. 큰 타입을 작은 타입 안에 저장할 때 주의해야 합니다.
long l = long.MaxValue; // long의 가장 큰 값 저장
// l 출력 : 9223372036854775807
int i = (int) l;
// i 출력 : -1
// int 값의 범위를 벗어나, 잘못된 값이 저장되었습니다.
Convert 클래스로 형식 변환
double d = 12.34;
int i = 1234;
d = i;
// d 출력 : 1234
d = 12.34;
i = (int) d;
// i 출력 : 12
string s = "";
s = Convert.ToString(d);
// s 출력 : 12.34
문자열을 정수 형식으로 변환하는 세 가지 방법
Convert.ToInt32(str);
int.Parse(str);
Int32.Parse(str);
TryParse로 안전하게 형변환
using System;
public class Example
{
public static void Main()
{
string[] values = { null, "160519", "9432.0", "16,667",
" -322 ", "+4302", "(100);", "01FA" };
foreach (var value in values)
{
int number;
bool success = int.TryParse(value, out number);
if (success)
{
Console.WriteLine($"Converted '{value}' to {number}.");
}
else
{
Console.WriteLine($"Attempted conversion of '{value ?? "<null>"}' failed.");
}
}
}
}
// The example displays the following output:
// Attempted conversion of '<null>' failed.
// Converted '160519' to 160519.
// Attempted conversion of '9432.0' failed.
// Attempted conversion of '16,667' failed.
// Converted ' -322 ' to -322.
// Converted '+4302' to 4302.
// Attempted conversion of '(100);' failed.
// Attempted conversion of '01FA' failed.
반응형
'C#' 카테고리의 다른 글
[C#] 참조 형식(Reference Types)과 값 형식(Value Types) (3) | 2022.09.10 |
---|---|
[C#] Immutable과 Mutable 타입 (0) | 2022.09.10 |
[C#] 박싱(Boxing)과 언박싱(Unboxing) (0) | 2022.09.10 |
[C#] 깊은 복사(Deep Copy)와 얕은 복사(Shallow Copy)의 이해 (0) | 2022.09.10 |
[C#] const와 readonly 차이 (0) | 2022.09.10 |
댓글